안녕하세요! "pulluper" 입니다.
이번 포스팅에서 다룰 주제는 ILSVRC(Imagenet) 에 대한 설명과 torchvision library 를 통한
Imagenet validation set 성능평가 입니다.
Detection 혹은 Network 관련 논문을 4개정도 뽑아서 논문에서 Imagenet 이라는 단어를 찾아봤을때
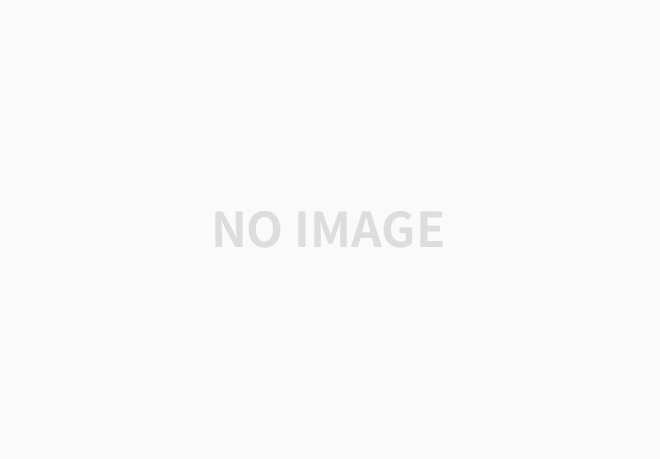
모두 ImageNet 에 대한 내용들이 있었습니다.
Imagenet 이란?
ILSVRC(ImageNet Large Scale Visual Recognition Challenge)
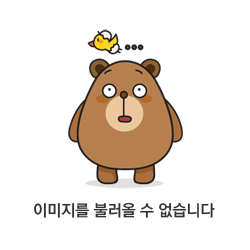
대표적인 large scale classification dataset 으로, 1000개의 class를 분류하는 분류 문제 dataset 입니다.
ImageNet
Research updates on improving ImageNet data
image-net.org
위의 사이트에서 매년 이미지 분류 대회를 열었는데, test 은 공개하고 있지 않고,
train 과 val set 을 얻을 수 있습니다.
train / validation data 의 크기는 다음과 같습니다.
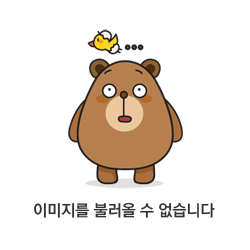
Dataset download
먼저 data를 다운로드 해 보겠습니다.
윈도우 기준이라 wget library 를 사용했습니다. (<--없으신 분들은 pip install wget 해주세요!)
download_imagenet 이라는 함수에서 root라는 parameter 는 data 를 받을 위치의 root directory 입니다.
import os
import wget
def bar_custom(current, total, width=80):
progress = "Downloading: %d%% [%d / %d] bytes" % (current / total * 100, current, total)
return progress
def download_imagenet(root='D:\data\example'):
"""
download_imagenet validation set
:param img_dir: root for download imagenet
:return:
"""
# make url
val_url = 'http://www.image-net.org/challenges/LSVRC/2012/dd31405981ef5f776aa17412e1f0c112/ILSVRC2012_img_val.tar'
devkit_url = 'http://www.image-net.org/challenges/LSVRC/2012/dd31405981ef5f776aa17412e1f0c112/ILSVRC2012_devkit_t12.tar.gz'
print("Download...")
os.makedirs(root, exist_ok=True)
wget.download(url=val_url, out=root, bar=bar_custom)
print('')
wget.download(url=devkit_url, out=root, bar=bar_custom)
print('')
print('done!')
위 함수를 실행하면 다운로드가 진행됩니다.
ILSVRC2012_img_val.tar
ILSVRC2012_devkit_t12.tar.gz 를 받게 됩니다.
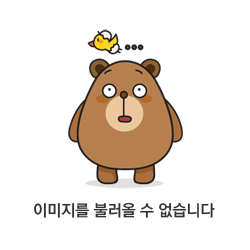
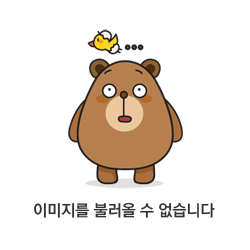
이렇게 data 를 다운받게 되면 내가 원하는 폴더안에 다음과 같은 파일들이 생성됩니다.
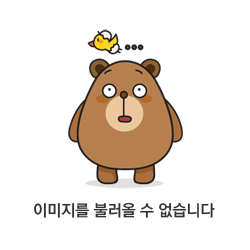
+240412)
현재는 위의 코드는 실행이 되지 않고, Imagenet 공식 사이트에서 로그인을 하고 데이터를 받을 수 있게 되었습니다~
Dataset
torchvision 에는 고맙게도 이미지넷을 위한 dataset 을 제공해주고 있습니다.
다만 이전에 다운받은 2가지의 file 들이 필요합니다.
자세한 사항은 다음을 참고하시면 됩니다.
pytorch.org/vision/0.8/datasets.html#imagenet
torchvision.datasets — Torchvision 0.8.1 documentation
torchvision.datasets All datasets are subclasses of torch.utils.data.Dataset i.e, they have __getitem__ and __len__ methods implemented. Hence, they can all be passed to a torch.utils.data.DataLoader which can load multiple samples parallelly using torch.m
pytorch.org
import torchvision
testset = torchvision.datasets.ImageNet(root="D:\data\example", split='val')
이제 위와같은 코드 단 한줄로 pytorch 에서 사용할 dataset 이 만들어졌습니다.
root는 아까 download 받은 파일들이 존재하는 폴더여야 합니다.
이 코드를 실행하면 잠시 시간이 흐른후 그 root directory 에 다음과 같은 파일들이 더 생깁니다.
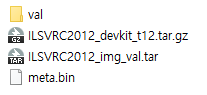
Model
여기서 사용할 모델은 torchvision 에서 제공하는 다음과 같은 모델들은 모두 사용 할 수 있습니다.
물론 pretrained 된 weight 도 사용가능합니다.
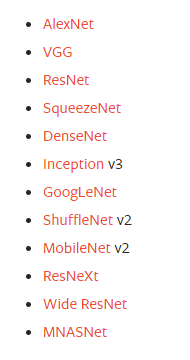
pytorch.org/vision/0.8/models.html
torchvision.models — Torchvision 0.8.1 documentation
torchvision.models The models subpackage contains definitions of models for addressing different tasks, including: image classification, pixelwise semantic segmentation, object detection, instance segmentation, person keypoint detection and video classific
pytorch.org
우리는 다음과 같은 2012년 ILSVRC 대회에서 우승을 차지한 alexnet 을 사용해 보도록 하겠습니다.
deep neural network 의 시작을 알리는 네트워크 라고 할수 있죠.
다음과 같은 코드로 model 설정이 완료가 되었습니다! 또한 torchvision 은 또 고맙게도 image train dataset 으로
미리 train 한 (pretrained=True) 인 option 까지 제공합니다.
from torchvision.models import alexnet
model = alexnet(pretrained=True)
Evaluation
dataset 에서 transform 이나 normalization 등의 부분은 imagenet 의 setting 입니다.
import torch
import torchvision
import torch.utils.data as data
import torchvision.transforms as transforms
if __name__ == "__main__":
device = torch.device('cuda:0' if torch.cuda.is_available() else 'cpu')
normalize = transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
transform = transforms.Compose(
[transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
normalize,
])
test_set = torchvision.datasets.ImageNet(root="D:\Data\example", transform=transform, split='val')
test_loader = data.DataLoader(test_set, batch_size=100, shuffle=True, num_workers=4)
model = torchvision.models.alexnet(pretrained=True).to(device)
model.eval()
correct_top1 = 0
correct_top5 = 0
total = 0
with torch.no_grad():
for idx, (images, labels) in enumerate(test_loader):
images = images.to(device) # [100, 3, 224, 224]
labels = labels.to(device) # [100]
outputs = model(images)
# ------------------------------------------------------------------------------
# rank 1
_, pred = torch.max(outputs, 1)
total += labels.size(0)
correct_top1 += (pred == labels).sum().item()
# ------------------------------------------------------------------------------
# rank 5
_, rank5 = outputs.topk(5, 1, True, True)
rank5 = rank5.t()
correct5 = rank5.eq(labels.view(1, -1).expand_as(rank5))
# ------------------------------------------------------------------------------
for k in range(6):
correct_k = correct5[:k].view(-1).float().sum(0, keepdim=True)
correct_top5 += correct_k.item()
print("step : {} / {}".format(idx + 1, len(test_set)/int(labels.size(0))))
print("top-1 percentage : {0:0.2f}%".format(correct_top1 / total * 100))
print("top-5 percentage : {0:0.2f}%".format(correct_top5 / total * 100))
print("top-1 percentage : {0:0.2f}%".format(correct_top1 / total * 100))
print("top-5 percentage : {0:0.2f}%".format(correct_top5 / total * 100))
결과는 다음과 같습니다.

따라서 error rate 각각 43.48 / 20.93 입니다. 그런데, torchvision 에서 제시한 수치와 약간의 차이가 있었습니다.
(약 0.03 / 0.02)

이와 관련된 문제는 다음 사이트에서 찾을 수 있었는데요.
github.com/pytorch/vision/issues/3152
Small discrepancy in accuracy & Some results of pretrained classifiers are missing in doc · Issue #3152 · pytorch/vision
📚 Documentation With torch==1.7.0 and torchvision==0.8.1, I found small discrepancy in accuracy (doc vs. code) pretrained ShuffleNet V2 (x0.5) and MNASNet 0.5 are available, but the numbers are mis...
github.com
"불일치가 매우 작다는 점을 감안할 때 이것이 코드 변경의 결과인지 아니면 인프라와 귀하의 설정 (GPU 수,
ImageNet 사본 등)의 차이로 인한 것인지는 확실하지 않습니다. 혹은 lua-torch 에서 이식될때, 차이가 날
수 있습니다" 라고 대답하고 있습니다.
torchvision 공식 사이트의 몇가지 network accuracy 들과 비교해 본 표입니다.
거의 같거나 0.04 이내의 차이를 나타내고 있습니다.
Network | Top-1 error | Top-5 error | (Our) Top-1 error | (Our) Top-5 error |
Alexnet | 43.45 | 20.91 | 43.48 (+0.03) | 20.93 (+0.02) |
VGG-11 | 30.98 | 11.37 | 30.98 | 11.37 |
VGG-13 | 30.07 | 10.75 | 30.07 | 10.75 |
VGG-16 | 28.41 | 9.62 | 28.41 | 9.62 |
VGG-19 | 27.62 | 9.12 | 27.62 | 9.12 |
VGG-11 bn | 29.62 | 10.19 | 29.63 (+0.01) | 10.19 |
VGG-13 bn | 28.45 | 9.63 | 28.41 (-0.04) | 9.63 |
VGG-16 bn | 26.63 | 8.50 | 26.64 (+0.01) | 8.48 (-0.02) |
VGG-19 bn | 25.76 | 8.15 | 25.78 (+0.02) | 8.16 (+0.01) |
ResNet-18 | 30.24 | 10.92 | 30.24 | 10.92 |
ResNet-34 | 26.70 | 8.58 | 26.69 (-0.01) | 8.58 |
ResNet-50 | 23.85 | 7.13 | 23.87 (+0.02) | 7.14 (+0.01) |
ResNet-101 | 22.63 | 6.44 | 22.63 | 6.45 (+0.01) |
ResNet-152 | 21.69 | 5.94 | 21.69 | 5.95 (+0.01) |
ResNeXt-50-32x4d | 22.38 | 6.30 | 22.38 | 6.30 |
ResNeXt-101-32x8d | 20.69 | 5.47 | 20.69 | 5.47 |
Wide ResNet-50-2 | 21.49 | 5.91 | 21.53 (+0.04) | 5.91 |
Wide ResNet-101-2 | 21.16 | 5.72 | 21.15 (-0.01) | 5.72 |
+ 2021.08.30 추가
파이토치 models 에 성능이 다시 업데이트가 되었습니다. 성능들이! 오른쪽과 같네요 :)
https://pytorch.org/vision/stable/models.html
torchvision.models — Torchvision 0.10.0 documentation
torchvision.models The models subpackage contains definitions of models for addressing different tasks, including: image classification, pixelwise semantic segmentation, object detection, instance segmentation, person keypoint detection and video classific
pytorch.org
Result
여기까지 잘 따라오셨다면, 여러분들은 torchvision 을 이용해서 간단히 imagenet 의 pretrained model 을 classification
에 활용 할 수 있습니다! 이제 pretrained model 이 필요한 모델링에서 한층 더 쉽게 접근 하실 수 있을 것입니다.
잘못된 점이나 질문은 항상 환영합니다.
감사합니다. :)
출처 :
cloud.google.com/tpu/docs/imagenet-setup?hl=nl
Downloading, pre-processing, and uploading the ImageNet dataset
This topic describes how to download, pre-process, and upload the ImageNet dataset to use with Cloud TPU. Machine learning models that use the ImageNet dataset include: ResNet AmoebaNet EfficientNet MNASNet ImageNet is an image database. The images in the
cloud.google.com
cv.gluon.ai/build/examples_datasets/imagenet.html
Prepare the ImageNet dataset — gluoncv 0.10.0 documentation
cv.gluon.ai
github.com/pytorch/examples/tree/master/imagenet
pytorch/examples
A set of examples around pytorch in Vision, Text, Reinforcement Learning, etc. - pytorch/examples
github.com
'Network' 카테고리의 다른 글
[DNN] VGG구현을 위한 리뷰(ICLR 2015) (0) | 2022.07.27 |
---|---|
[DNN] ViT 구조 (0) | 2022.06.08 |
[DNN] Alexnet 리뷰 및 구현 (NIPS 2012) (0) | 2021.09.24 |
[DNN] U-net 구조와 code 구현 (MICCAI2015) (4) | 2021.05.02 |
[DNN] Densenet 논문리뷰 및 구현 (CVPR2017) (0) | 2021.04.13 |
댓글